Chapter 3. Identity Management - Overview
3.1. Introduction
PicketLink Identity Management (IDM) is a fundamental module of PicketLink, with all other modules building on top of the IDM component to implement their extended features. It features provide a rich and extensible API for managing the identities (such as users, groups and roles) of your applications and services. It also supports a flexible system for identity partitioning, allowing it to be used as a complete security solution in simple web applications and/or as an Identity Provider (IDP) in more complex multi-domain scenarios. It also provides the core Identity Model API classes (see below) upon which an application's identity classes are defined to provide the security structure for that application.
The Identity Management features of PicketLink are accessed primarily via the following three interfaces:
-
PartitionManager
is used to manage identity partitions, which are essentially a container for a set of identity objects. ThePartitionManager
interface provides a set of CRUD methods for creating, reading, updating and deleting partitions, as well as methods for creating anIdentityManager
orRelationshipManager
(more on these next). A typical Java EE application with simple security requirements will likely not be required to access thePartitionManager
API directly. -
IdentityManager
is used to manage identity objects within the scope of a partition. Some examples of identity objects are users, groups and roles, although PicketLink is not limited to just these. Besides providing the standard set of CRUD methods for managing and locating identity objects, theIdentityManager
interface also defines methods for managing credentials and for creating identity queries which may be used to locate identities by their properties and attributes. -
RelationshipManager
is used to manage relationships - a relationship is a typed association between two or more identities, with each identity having a definitive meaning within the relationship. Some examples of relationships that may already be familiar are group memberships (where a user is a member of a particular group) or granted roles (where a user is assigned to a role to afford them a certain set of privileges). TheRelationshipManager
provides CRUD methods for managing relationships, and also for creating a relationship query which may be used to locate relationships between identities based on the relationship type and participating identity object/s. -
PermissionManager
is used to manage permissions. For more details take a look at Chapter 10, Identity Management - Permissions API and Permission Management.
Note
In case you are wondering why a separate
RelationshipManager
interface is required for managing relationships between identites, it is because PicketLink supports relationships between identities belonging to separate partitions; therefore the scope of a RelationshipManager
instance is not constrained to a single partition in the same way as the IdentityManager
.
Interaction with the backend store that provides the persistent identity state is performed by configuring one or more
IdentityStore
s. PicketLink provides a few built-in IdentityStore
implementations for storing identity state in a database, file system or LDAP directory server, and it is possible to provide your own custom implementation to support storing your application's identity data in other backends, or extend the built-in implementations to override their default behaviour.
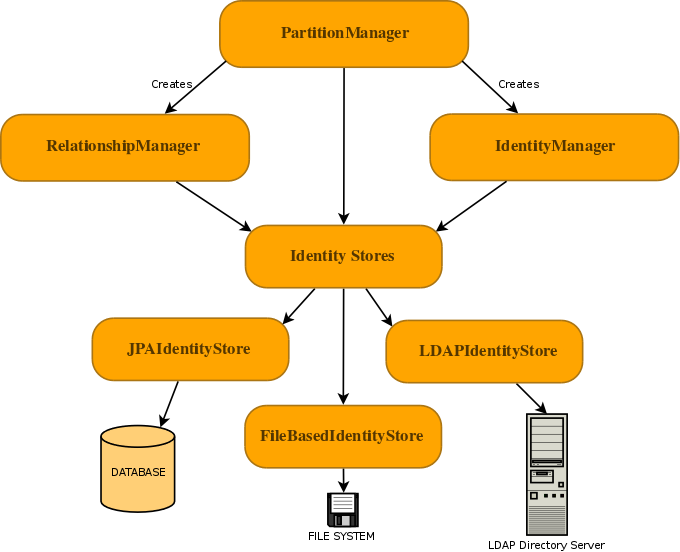
3.1.1. Injecting the Identity Management Objects
In a Java EE environment PicketLink provides a set of producer methods for injecting the primary identity management objects into your CDI beans. The following table outlines which IDM classes may be injected, and the CDI scope of each of the beans.
Table 3.1. Identity Management Objects
Class Name | Scope |
---|---|
org.picketlink.idm.PartitionManager | @ApplicationScoped |
org.picketlink.idm.IdentityManager | @RequestScoped |
org.picketlink.idm.RelationshipManager | @RequestScoped |
org.picketlink.idm.PermissionManager | @RequestScoped |
3.1.2. Interacting with PicketLink IDM During Application Startup
Since the
IdentityManager
and RelationshipManager
beans are request scoped beans (as per the above table) it is not possible to inject them directly into a @Startup
bean as there is no request scope available at application startup time. Instead, if you wish to use the IDM API within a @Startup
bean in your Java EE application you may inject the PartitionManager
(which is application-scoped) from which you can then get references to the IdentityManager
and RelationshipManager
:
@Singleton @Startup public class InitializeSecurity { @Inject private PartitionManager partitionManager; @PostConstruct public void create() { // Create an IdentityManager IdentityManager identityManager = partitionManager.createIdentityManager(); User user = new User("shane"); identityManager.add(user); Password password = new Password("password"); identityManager.updateCredential(user, password); // Create a RelationshipManager RelationshipManager relationshipManager = partitionManager.createRelationshipManager(); // Create some relationships } }
3.1.3. Configuring the Default Partition
Since PicketLink has multiple-partition support, it is important to be able to control the associated partition for the injected
IdentityManager
.
By default, PicketLink will inject an
IdentityManager
for the default Realm (i.e. the org.picketlink.idm.model.basic.Realm
partition with a name of default). If your application has basic security requirements then this might well be adequate, however if you wish to override this default behaviour then simply provide a producer method annotated with the @PicketLink
qualifier that returns the default partition for your application:
@ApplicationScoped public class DefaultPartitionProducer { @Inject PartitionManager partitionManager; @Produces @PicketLink public Partition getDefaultPartition() { return partitionManager.getPartition(Realm.class, "warehouse.dispatch"); } }
Warning
Before producing a partition, make sure it was already created. For most cases, PicketLink will always create the default partition during startup. But if you want to produce different ones, you must create them manually. Please, take a look at Section 3.7, “Partition Management” for more details about how to manage partitions.
This feature is also very useful if you are dealing with multiple partitions and if you want to choose between them dynamically. In this case, you can have a producer that decides which
Partition
to produce based on a condition. For instance, you can create conditions based on the user`s name (eg.: if they use an email to login, you can choose the partition on a per-domain basis), an input from a page or whatever other information available from the bean producing partitions.