Chapter 5. Identity Management - Basic Identity Model
5.1. Basic Identity Model
For the sake of convenience, PicketLink provides a basic identity model that consists of a number of core interfaces which define a set of fundamental identity types which might be found in a typical application. The usage of this identity model is entirely optional; for an application with basic security requirements the basic identity model might be more than sufficient, however for a more complex application or application with custom security requirements it may be necessary to create a custom identity model.
The following class diagram shows the classes and interfaces in the
org.picketlink.idm.model.basic
package:
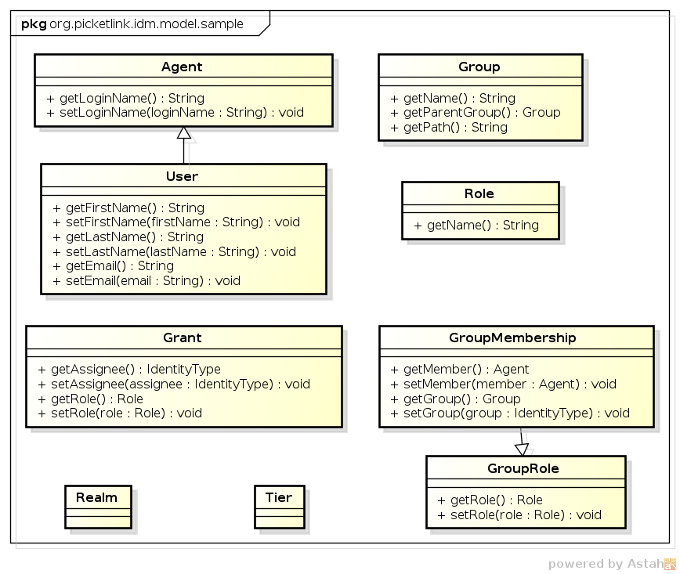
-
Agent
represents a unique entity that may access the services secured by PicketLink. In contrast to a user which represents a human,Agent
is intended to represent a third party non-human (i.e. machine to machine) process that may authenticate and interact with your application or services. It declares methods for reading and setting theAgent
's login name. -
User
represents a human user that accesses your application and services. In addition to the login name property defined by its parent interfaceAgent
, theUser
interface declares a number of other methods for managing the user's first name, last name and e-mail address. -
Group
is used to manage collections of identity types. EachGroup
has a name and an optional parent group. -
Role
is used in various relationship types to designate authority to another identity type to perform various operations within an application. For example, a forum application may define a role called moderator which may be assigned to one or moreUser
s orGroup
s to indicate that they are authorized to perform moderator functions. -
Grant
relationship represents the assignment of aRole
to an identity. -
GroupMembership
relationship represents aUser
(orAgent
) membership within aGroup
. -
GroupRole
relationship represents the the assignment of a specificRole
within aGroup
to aUser
orAgent
. The reason this relationship extends theGroupMembership
relationship is simply so it inherits thegetMember()
andgetGroup()
methods - being assigned to aGroupRole
does not mean that theUser
(orAgent
) that was assigned the group role also becomes a member of the group. -
Realm
is a partition type, and may be used to store anyIdentityType
objects (includingAgent
s,User
s,Group
s orRole
s. -
Tier
is a specialized partition type, and may be only used to storeGroup
orRole
objects specific to an application.
5.1.1. Utility Class for the Basic Identity Model
PicketLink also provides an utility class with some very useful and common methods to manipulate the basic identity model. Along the documentation you'll find a lot of examples using the the following class:
org.picketlink.idm.model.basic.BasicModel
. If you're using the basic identity model, this helper class can save you a lot of code and make your application even more simple.
The list below summarizes some of the functionalities provided by this class:
-
Retrieve
User
andAgent
instances by login name. -
Retrieve
Role
andGroup
instances by name. -
Add users as group members, grant roles to users. As well check if an user is member of a group or has a specific role.
One import thing to keep in mind is that the
BasicModel
helper class is only suitable if you're using the types provided by the basic identity model, only. If you are using custom types, even if those are sub-types of any of the types provided by the basic identity model, you should handle those custom types directly using the PicketLink IDM API.
As an example, let's suppose you have a custom type which extends the
Agent
type.
SalesAgent salesAgent = BasicModel.getUser(identityManager, "someSalesAgent");
The code above will never return a
SalesAgent
instance. The correct way of doing that is using the Query API directly as follows:
public SaleAgent findSalesAgent(String loginName) { IdentityQueryBuilder queryBuilder = identityManager.getQueryBuilder(); List<SaleAgent> result = queryBuilder .createIdentityQuery(SaleAgent.class) .where(queryBuilder.equal(SaleAgent.LOGIN_NAME, loginName)) .getResultList(); return result.isEmpty() ? null : result.get(0); }
Warning
Please note that the
BasicModel
helper class is only suitable for use cases where only the types provided by the basic identity model are used. If your application have also custom types, they need to be handled directly using the PicketLink IDM API.